Cryptography
Understanding Bagel's cryptographic implementation
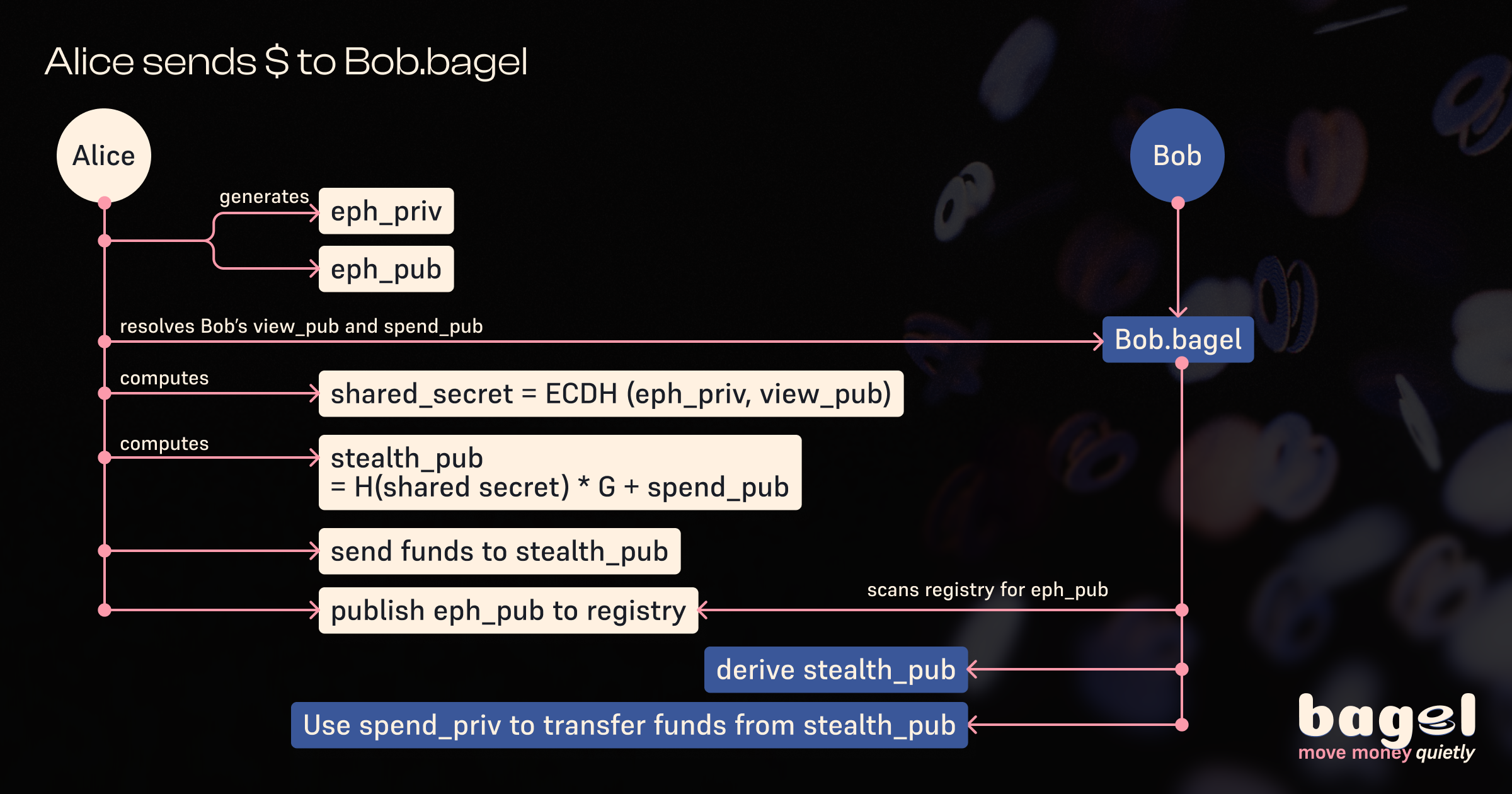
Bagel uses advanced cryptography to provide privacy and security for your transactions. This document explains the key cryptographic functions and processes used in Bagel.
Key Types and Derivation
Viewing and Spending Keys
The core of Bagel's privacy model is the separation of viewing and spending keys:
- Viewing Keys: Used to discover and identify stealth payments sent to you
- Spending Keys: Used to control and spend funds from stealth addresses
Both keys are derived deterministically from your wallet signature:
Ephemeral Keys
For each transaction, a new ephemeral key pair is generated:
Key Exchange and Shared Secrets
Bagel uses X25519 Diffie-Hellman key exchange to create shared secrets:
Stealth Address Creation
The core privacy feature of Bagel is the creation of unique stealth addresses for each transaction:
Stealth Address Recovery
Recipients can recover stealth addresses sent to them using their keys:
This function:
- Validates the spending key pair
- Creates the same tag as in stealth address creation
- Derives the same stealth keypair using identical HMAC-SHA256 parameters
- Returns the reconstructed keypair, which can access funds sent to the stealth address
Message Signing and Verification
Bagel includes utilities for signing and verifying messages:
Data Encryption and Decryption
In addition to stealth address functionality, Bagel provides utilities for general data encryption:
Libraries and Standards
Bagel uses the following cryptographic libraries and standards:
- Noble Curves (
@noble/curves
) - For Ed25519 and X25519 operations - Noble Hashes (
@noble/hashes
) - For SHA-256, HMAC, and utility functions - Web Crypto API - For encryption operations (AES-GCM, PBKDF2)
- Solana Web3.js - For Solana-specific keypair operations
All cryptographic operations follow industry best practices for secure implementation.